Set up Timers
As an application designer or story developer, you can use the timer technical object with script APIs to trigger timing events in view time.
Context
-
Creating simple animations that make image or text widgets change their contents every couple of seconds
-
Sending notifications to end users at a certain interval
-
Refreshing analytic applications or optimized stories at a certain interval
Multiple timers can exist simultaneously, but each timer works independently from others.
Procedure
Example
Here's an example of using the timer to automatically scroll images horizontally from right to left at a certain interval.
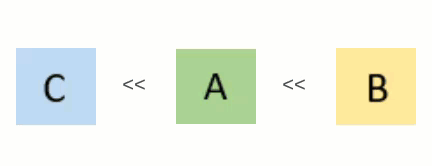
First, insert three different images, Image_1, Image_2 and Image_3 and place them side by side on the page. Take notes of their left margins, which are 112px, 240px and 368 px.
Then, create an image type script variable Animation_Widgets and an integer type script variable LeftMargins. Enable Set As Array for the two script variables.
After that, add a script object Util_Animation with a function doAnimation and then add a timer Timer_1 .
Write the following scripts:
For the onInitialization event of page:
console.log('onInitialization - begin'); LeftMargins = [112, 240, 368]; Animation_Widgets = [Image_1,Image_2,Image_3]; //Start the timer after 3 seconds after initializing the page. Timer_1.start(3); console.log('onInitialization - end');
For doAnimation:
var num = 3; //Redefine the sequence of the images. var first = Animation_Widgets[0]; Animation_Widgets[0] = Animation_Widgets[1]; Animation_Widgets[1] = Animation_Widgets[2]; Animation_Widgets[2] = first; // Update the left margin of each widget. for(var i=0;i<num;i++) { Animation_Widgets[i].getLayout().setLeft(LeftMargins[i]); }
For Timer_1:
//Run this script object once Timer_1 times out. Util_Animation.doAnimation(); //Restart Timer_1 again after 3 seconds. Timer_1.start(3);
When end users view the application or story, they can soon see the images automatically scroll from right to left in turn.