Use Bookmark Set Technical Objects in Analytic Applications
As an
application designer, you can use a bookmark set technical object to let application users save the state of your analytic application
as a bookmark at runtime.
Procedure
-
In the Scripting section of Outline, select
right next to
Bookmark Set.
The side panel Bookmark Set opens, with the default name of the bookmark BookmarkSet_1
displayed. You can change the name if you want to.
-
Enter a version number. The number must be an integer.
Note
To generate a new version of the bookmark set at any time, change the version number. After that, all the bookmarks created based on the
previous version will become invalid. To reopen the invalid bookmarks, you need to change the version number back to the
previous one.
-
Select Included Components to choose the canvas, popups, widgets and technical objects to be included in
bookmarks.
-
Select Done to finish configuring the bookmark set technical object.
-
To let application users save a bookmark including its properties when viewing the analytic application, you need to add a widget such as a
button and write script for it, using the syntax below:
Code Syntax
// Save bookmark contents with information such as name and properties in key-value pair
saveBookmark(BookmarkSaveInfo: BookmarkSaveInfo, overwrite?: boolean): BookmarkInfo
//BookmarkSaveInfo contains "name"(mandatory), "isGlobal", "isDefault", "properties" and "isKeepLastDynamicVariableValue" as objects keys.
//BookmarkInfo contains "id", "name", "displayName", "version", "isGlobal", "isDefault", and "properties" as objects keys.
Note
You can define whether application users’ last changes to the dynamic variable values can be saved with bookmarks via API or
Analytic Application Details. If application user saves a bookmark when you haven’t enabled the
option, it might be opened with the last changed variable values of the last opened bookmark after you later set
isKeepLastDynamicVariableValue? to true or selected Override default
settings and Keep last saved values for dynamic variables.
You can also leverage the following APIs to let application users apply, list, delete bookmarks or open the bookmark sharing dialog in the
application:
Code Syntax
apply(bookmark: string | BookmarkInfo): boolean // Apply bookmark to the current application.
getAll(): BookmarkInfo[] // Return all valid bookmarks of current application.
getAppliedBookmark(): BookmarkInfo // Return the bookmark which is applied to current application.
getVersion(): integer // Return current bookmark version of application.
deleteBookmark(bookmark: string | BookmarkInfo): boolean // Delete specified bookmark of application.
openShareBookmarkDialog(bookmarkId : string) : void // Open the bookmark sharing dialog.
-
Save and run the analytic application.
Results
After creating a bookmark set technical object and writing the scripts for it, you can let application users
change the states of the widgets at will, save different states as bookmarks and view them at any time.
Example
Here are some script examples that show how to let application users save, list and delete bookmarks in runtime by leveraging bookmark
related APIs.
Example
In this example, you want to design an application that lets application users capture the application state and save it
as a bookmark with property.
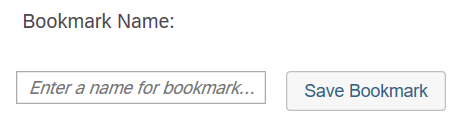
First, in addition to the technical object
BookmarkSet_1, add an input field InputField_1 and a button
Button_1 to the canvas.
Then write the following script for Button_1:
Sample Code
var bkName = InputField_1.getValue();
BookmarkSet_1.saveBookmark({name: bkName, isGlobal: true, properties: {"modelId": “BestRunJuice”}}, true);
Via this script, you can create a global bookmark with the name entered in the input field, and the existing bookmarks will be
overridden if the name duplicates. It also allows you to add an additional property modelID with
BestRunJuice as value.
Example
In this example, you want to let application users remove any bookmarks in the application that are all listed in the
dropdown.
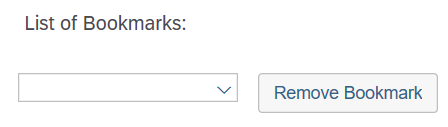
First, in addition to the technical
object BookmarkSet_1, add a dropdown Dropdown_1 and another button
Button_2 to the canvas.
Then write the following script for the widgets:
For
Dropdown_1:
Sample Code
var bookmarks = BookmarkSet_1.getAll();
Dropdown_1.removeAllItems();
for (var i=0; i < bookmarks.length;++i)
{
Dropdown_1.addItem(bookmarks[i].id, bookmarks[i].name);
}
if (bookmarks.length > 0)
{
Dropdown_1.getSelectedKey();
}
For Button_2:
Sample Code
BookmarkSet_1.deleteBookmark(Dropdown_1.getSelectedKey());
When application users open the dropdown list, they can see all the available bookmarks. Then, when selecting
Remove Bookmark, they can delete the bookmarks selected from the dropdown.