Use Pause Refresh Options and APIs
In edit time, as an application designer or story developer, you can configure data refresh and interaction settings of specific charts or tables in the Builder panel or via script APIs to improve the efficiency of retrieving and updating data after the widgets're initially loaded in view time.
Currently, R visualization widgets aren't supported for the settings.
Modes of Data Refresh
- Always Refresh (Default)
- Refresh Active Widgets Only: Under this mode, you can avoid
unnecessary data refresh. Visible widgets refresh, while data refresh for invisible widgets is automatically paused except when
they're called by specific fetch data script APIs.:
DataSource.getResultSet, DataSource.getData, DataSource.isResultEmpty, DataSource.getResultMember, DataSource.getDataSelections, DataSource.expandNode, DataSource.refreshData
Table.getColumnCount, Table.getRowCount
Query is sent by tables even if the latest data’s been fetched, but won’t be sent by charts.
NoteWidgets in inactive popup dialogs, tabs in tab strips and pages in page books are treated as invisible ones.
In addition, onResultChanged event is still triggered when the result set from an invisible widget is updated by script APIs such as setDimensionFilter and setVariableValue.
When invisible widgets become visible, any previously paused operations that result in data refresh impact them again and update their data. Query is sent by tables whether data are changed or not.
-
Always Pause: No data refresh takes place in view time, including initial loading.
We recommend selecting Disable Interaction as most of the interactions won’t take effect.
Later when you disable this mode, DataSource.refreshData and other refresh activities can be automatically resumed except for planning activities, which needs to be manually triggered.
Script APIs
You can also leverage DataSource.setRefreshPaused API to let end users set the data refresh mode in view time. The script is fully executed without waiting for all the widgets associated with the data source to be updated when their pause mode isn't on. The supported widgets are charts and tables.
// Set the pause mode of the data refresh. DataSource.setRefreshPaused(paused: PauseMode | boolean): void
// Get data refresh mode, which returns On, Off or Auto. DataSource.getRefreshPaused(): PauseMode
The modes defined in script APIs correspond to the options in the Builder panel:
In Script APIs | In Builder Panel |
---|---|
PauseMode.On | Always Pause |
PauseMode.Off | Always Refresh |
PauseMode.Auto | Refresh Active Widgets Only |
You can also use ApplicationPage.setRefreshPaused (in Optimized Story Experience) and Application.setRefreshPaused to enable or disable data refresh on page, story or application levels in view time. When the pause of data refresh is disabled, the widgets associated with the data sources are updated at the same time with data refresh.
setRefreshPaused(dataSources: DataSource[], paused: boolean): void
If you set data refresh mode for the same widget in both Builder panel and script editor, scripting will overwrite the settings in the panel.
When planning is enabled, viewers can't pause a widget via setRefreshPaused API. They need to disable planning first via setPlanningEnabled API.
Influence on Other Functionalities When Widgets are in Pause Mode
-
Refresh in the toolbar in view time won’t work on this widget.
-
All context menus (including right-click menu and quick action menu) are disabled.
-
All data related actions that can trigger query are disabled.
-
Planning is automatically disabled, which means:
-
In edit time, Planning Enabled in the widget’s Builder panel is automatically disabled. If the widget is later set to always refresh, the Planning Enabled option becomes available and is selected by default.
-
In view time, planning is disabled. If the widget is later set to always refresh via API, planning won’t be resumed automatically. It needs to be enabled via setPlanningEnabled API.
-
If viewers change to another planning model, planning is still automatically disabled.
-
Changing planning data in another table from the same planning model won’t trigger data refresh in the table in pause mode.
-
All planning APIs (including ones that enable planning) won’t take effect or return default value according to its return type declaration (except for submitData, setUserInput and setPlanningEnabled, which return false instead).
-
Viewers can enable planning for tables in pause mode. However, they will receive a warning message that they can't change to PauseMode.On or PauseMode.Auto again once planning is enabled. To prevent them from enabling planning and losing planning data they input, we recommend you to disable planning before enabling widgets to pause.
-
-
Changing script variables used in calculated measure won’t result in the refresh of related widgets.
-
PDF exporting is based on currently rendered result.
-
All modifications of time series forecast, smart grouping and navigation panel are applied to the widget’s existing state.
-
All APIs can be called, but won’t trigger data refresh of the widget, which means:
-
Application.refresh and DataSource.refreshData won’t work on the widget.
-
All Getter APIs will return results based on the widget’s existing state.
-
All Setter APIs will apply to the widget’s existing state and no data refresh is triggered.
NoteHowever, some local property and styling setting APIs such as DataSource.setMemberDisplay can cause the widget to re-render.
-
Open Explorer API and Smart Insights use the current state of the widget.
NoteHowever, pause mode doesn’t work on this widget in Explorer.
-
Prompt APIs can be called but the widget won’t refresh after prompt values are set.
-
SearchToInsight result API can be called but the widget won’t refresh.
-
-
If no values are defined for mandatory variables in a widget’s data source, the variable dialog pops up, for example, when the table in pause mode is called by setModel API.
Exceptional Cases When Widgets are in Pause Mode
There’re exceptional cases where some queries are still triggered even when widgets don't always refresh:
APIs | Queries (First Time Query Without Cache) |
---|---|
DataSource.getMembers | Query remote dimension members. |
DataSource.setDimensionFilter | Fetch member information if no MemberInfo object is specified in the API. Note
Therefore, we recommend you set MemberInfo if possible like the example below: Chart_1.getDataSource().setDimensionFilter("Location_4nm2e04531", { dimensionId: "Location_4nm2e04531", id: "[Location_4nm2e04531].[State_47acc246_4m5x6u3k6s].&[SA1]", description: "California", displayId: "California" }); |
DataSource.setVariableValue |
Note
This is applicable when variable is set via scripting in view time or initial value is set in edit time. |
DataSource.setHierarchy |
For SAP BW model, two queries are triggered:
|
DataSource.expandNode |
For SAP BW model, two queries are triggered:
|
DataSource.collapseNode |
For chart in SAP BW model, two queries are triggered:
|
Table.setModel Table.openSelectModelDialog |
For SAP BW model, five queries are triggered:
For other models, two queries are triggered:
|
Initial loading of application or story with SAP BW model and variables |
Two queries are triggered:
For table, extra four queries are triggered:
|
Example
Let’s say you want to let end users turn on or off data refresh and interaction of Chart_1 and Table_1 via two switches, Switch_Refresh and Switch_Interaction.
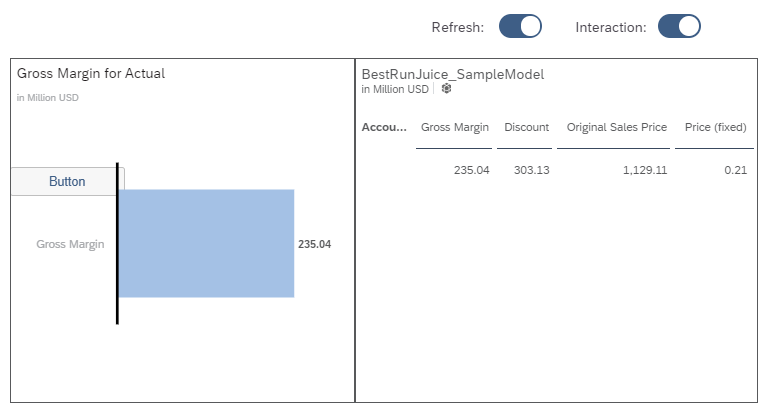
First, leave the options Always Refresh selected and Disable Interaction unselected in the Builder panel of both Chart_1 and Table_1. Meanwhile, both switches’ default status is on.
Write the following scripts for Switch_Refresh:
if (Switch_1.isOn()) { Chart_1.getDataSource().setRefreshPaused(PauseMode.Off); Table_1.getDataSource().setRefreshPaused(PauseMode.Off); } else { Chart_1.getDataSource().setRefreshPaused(PauseMode.On); Table_1.getDataSource().setRefreshPaused(PauseMode.On); }
Write the following scripts for Switch_Interaction:
Chart_1.setEnabled(!Chart_1.isEnabled()); Table_1.setEnabled(!Table_1.isEnabled());
When viewers turn off the two switches, the chart and table won’t refresh when they perform various activities, such as adding filters to and setting hierarchy level of the chart and table, setting the variable value used by the reference line in the chart and collapsing the parent node in the table.
Once they turn on the two switches again, the chart and table automatically refresh and display the latest results.