Configure Bookmark Settings and Use Related APIs (Optimized Story Experience)
As a story developer, you can configure settings and use related APIs for viewers to bookmark the state of the story.
Procedure
-
From View section in the toolbar, select Left Side Panel to open
Outline.
-
Select
Bookmarks.
The Bookmarks side panel opens, where you can configure settings for viewers to bookmark the state of the story.
Bookmarks is also the name of the bookmark technical object, which can be reused in scripting.
-
Enter a version number, which must be an integer.
This option is also available to story designers.
Note
If you change the version, all the bookmarks created based on the previous version become invalid. To reopen the invalid
bookmarks, you need to change the version number back to the previous one.
-
Select the scope of bookmarks:
- State-Changed Components Only: Bookmarks only include the widgets, technical objects or script variables whose states
have been changed, such as the display of data, width and height and variable values.
- All Components in the Story: All the widgets, technical objects and script variables in the story are bookmarked,
whether their states have been changed or not.
- Selected Components Only: Only the selected widgets, technical objects, script variables, pages or popups are
bookmarked. You can select what to be included in bookmarks.
-
Select Done.
-
In addition, you can use related APIs to let story viewers save a bookmark including its properties by clicking a button, for
example:
Code Syntax
// Save bookmark contents with information such as name and properties in key-value pair
saveBookmark(BookmarkSaveInfo: BookmarkSaveInfo, overwrite?: boolean): BookmarkInfo
//BookmarkSaveInfo contains "name"(mandatory), "isGlobal", "isDefault", "properties" and "isKeepLastDynamicVariableValue" as objects keys.
//BookmarkInfo contains "id", "name", "displayName", "version", "isGlobal", "isDefault", and "properties" as objects keys.
Note
In addition to the API, you can define whether story viewers’ last changes to the dynamic variable values can be saved with bookmarks via
Story Details. For more information, see Story Preferences and Details.
If viewers save a bookmark when you haven’t enabled the option, it might be opened with the last changed variable values of
the last opened bookmark after you later set isKeepLastDynamicVariableValue? to true or
selected Override default settings and Keep last saved values for dynamic
variables.
You can also leverage the following APIs to let viewers apply, list, delete bookmarks or open the bookmark sharing dialog in the story:
Code Syntax
apply(bookmark: string | BookmarkInfo): boolean // Apply bookmark to the current story.
getAll(): BookmarkInfo[] // Return all valid bookmarks of current story.
getAppliedBookmark(): BookmarkInfo // Return the bookmark which is applied to current story.
getVersion(): integer // Return current bookmark version of story.
deleteBookmark(bookmark: string | BookmarkInfo): boolean // Delete specified bookmark of story.
openShareBookmarkDialog(bookmarkId : string) : void // Open the bookmark sharing dialog.
-
Save your story.
Example
Here are some script examples that show how to let story viewers save, list and delete bookmarks in view time.
Example
In this example, you want to let viewers capture the state of the story and save it as a bookmark with property in a quicker
way.
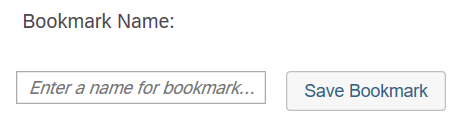
First, add an input field
InputField_1 and a button Button_1 to the story.
Then, write the following
script for Button_1:
Sample Code
var bkName = InputField_1.getValue();
Bookmarks.saveBookmark({name: bkName, isGlobal: true, properties: {"modelId": “BestRunJuice”}}, true);
Viewers can create a global bookmark with the name entered in the input field, and the existing bookmarks are overridden if the
name duplicates. An additional property modelID with BestRunJuice as value is saved with the
bookmark as well.
Example
In this example, you want to let story viewers remove any bookmarks of the story from the dropdown.
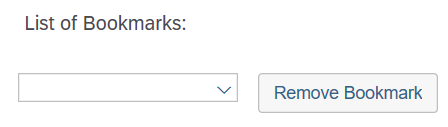
First, add a dropdown Dropdown_1
and another button Button_2 to the story.
Then write the following script for the widgets:
For
Dropdown_1:
Sample Code
var bookmarks = Bookmarks.getAll();
Dropdown_1.removeAllItems();
for (var i=0; i < bookmarks.length;++i)
{
Dropdown_1.addItem(bookmarks[i].id, bookmarks[i].name);
}
if (bookmarks.length > 0)
{
Dropdown_1.getSelectedKey();
}
For Button_2:
Sample Code
Bookmarks.deleteBookmark(Dropdown_1.getSelectedKey());
When story viewers open the dropdown list, they can see and select any available bookmarks. Then, when clicking
Remove Bookmark, they can delete the bookmarks selected from the dropdown.